| |
NOTE - Use your Browsers BACK Button to return to prior page or
CLICK here.
PARSE-Func - Put/Get Parsed Data |
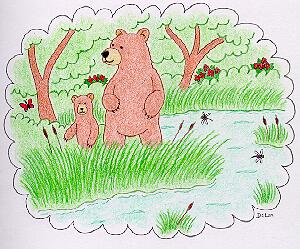 |
Here's a set of functions to Parse delimited files. It's based on a prior
page in my Techniques section (Parsing Delimited Files).
The functions are encapsulated for ease of use. We'll use these functions to produce a
solution to a recent question on the SQRUG list (07/21) - parsing a delimited record to
create XML output. Now check out the grizzly and then my moose (at the bottom). |

You can find the PARSE-Func functions in my custom SQR library TDFUNC.SQC

PARSE-Func-Put Parameters - Put
Fields Into Array (Parsing) |

PARSE-Func-Get - Parameters - Get
Parsed Field from Array |
 | Input - Index of Column to retrieve |
 | Output - Parsed Data Element (at Index Location) |
|

PARSE-Func-Put/Get in Action -
Parse Input and format XML... |
Here's the scenario: The user would like to read a semi-colon delimited
record which contains data in the following format:
Baker,Ron;972-972-9729;972-972-7297;;9727927@skytel.com;1200
The data elements include: Name, Home#, Page#, Cell#, E-mail and Work Ext.
The output should be produced in XML format as follows:
<PH>
<EMPLOYEE>Baker, Ron</EMPLOYEE>
<HOME_PHONE>972-972-9729</HOME_PHONE>
<PAGER>972-972-7297</PAGER>
<CELL></CELL>
<PAGER_EMAIL>9727927@skytel.com</PAGER_EMAIL>
<WORK_EXT>1200</WORK_EXT>
</PH>
Now we'll produce a quick little routine using PARSE-Func-Put (to parse the data) and
PARSE-Func-Get (to extract individual data elements). We'll use display commands to
produce the XML output in the example.
Here's the routine:
|
!**********************************************************************
!* Process Main *
!**********************************************************************
begin-procedure Process-Main
let #idx_name = 0
let #idx_home = 1
let #idx_page = 2
let #idx_cell = 3
let #idx_email = 4
let #idx_work = 5
while 1 = 1
read 1 into $rec:100
if #end-file = 1
break
end-if
do PARSE-Func-Put($rec, ';', '', #cols)
do PARSE-Func-Get(#idx_name, $I_name)
do PARSE-Func-Get(#idx_home, $I_home)
do PARSE-Func-Get(#idx_page, $I_page)
do PARSE-Func-Get(#idx_cell, $I_cell)
do PARSE-Func-Get(#idx_email, $I_email)
do PARSE-Func-Get(#idx_work, $I_work)
let $xml = '<PH>'
display $xml
let $xml = ' <EMPLOYEE>' || $I_name || '</EMPLOYEE>'
display $xml
let $xml = ' <HOME_PHONE>' || $I_home || '</HOME_PHONE>'
display $xml
let $xml = ' <PAGER>' || $I_page || '</PAGER>'
display $xml
let $xml = ' <CELL>' || $I_cell || '</CELL>'
display $xml
let $xml = ' <PAGER_EMAIL>' || $I_email || '</PAGER_EMAIL>'
display $xml
let $xml = ' <WORK_EXT>' || $I_work || '</WORK_EXT>'
display $xml
let $xml = '</PH>'
display $xml
end-while
end-procedure
!**********************************************************************
!* Include Members: *
!**********************************************************************
#Include 'tdfunc.sqc' !TD Custom SQR Function Library
...
...
!**********************************************************************
!* End of Program *
!**********************************************************************
|

!**********************************************************************
!* Build PARSE Array *
!**********************************************************************
!* *
!* See PARSE-Func-Put for String Parsing Parameters. *
!* See PARSE-Func-Get for Column Retrieval Parameters. *
!* *
!**********************************************************************
!* *
!* EXAMPLE: #define PARsize 5 ! Override Array Size *
!* ... *
!* let $rec = '123,"A,B,C",456' *
!* ... *
!* do PARSE-Func-Put($rec, ',', '"', #O_cols) *
!* ... *
!* do PARSE-Func-Get(0, $value1) *
!* do PARSE-Func-Get(1, $value2) *
!* do PARSE-Func-Get(2, $value3) *
!* *
!* RESULTS: <PARSE-Func-Put - Parsing> *
!* *
!* 3 in #O_cols variable (#columns parsed from $rec). *
!* *
!* Value 123 is placed in array element 0. *
!* Value A,B,C is placed in array element 1. *
!* Value 456 is placed in array element 2. *
!* *
!* Comma used as delimiter. *
!* Text Qualified with Double-Quotes. *
!* *
!* <PARSE-Func-Get - Individual Field Retrieval> *
!* *
!* $value1 set to array element 0 (123). *
!* $value2 set to array element 1 (A,B,C). *
!* $value3 set to array element 2 (456). *
!* *
!**********************************************************************
#ifndef PARSE_Func_Remove
#ifndef PARsize
#define PARsize 500 ! Default PARmtx Size
#endif
begin-procedure PARSE-Array
create-array name=PARmtx size={PARsize} field=PARfld:char
let #PARmax = {PARsize} - 1
end-procedure
!**********************************************************************
!* Parse Delimited Record (PUT Fields into Array) *
!**********************************************************************
!* *
!* INPUT: $I_rec - Input Record *
!* $I_del - Column Delimiter *
!* $I_txt - Text Qualifier (or NULL) *
!* OUTPUT: #O_cols - Output Number of Columns Loaded *
!* *
!* To de-activate this function: *
!* #define PARSE_Func_Remove *
!* *
!**********************************************************************
!* *
!* EXAMPLE: See PARSE-Array procedure for example. *
!* *
!**********************************************************************
begin-procedure PARSE-Func-Put($I_rec, $I_del, $I_txt, :#O_cols)
if #_PARmax = 0
do PARSE-Array
end-if
clear-array name=PARmtx
let $I_rec = $I_rec || $I_del
let #idx = 0
! Straight Delimiter Parsing (No Text Qualifiers Used)
if $I_txt = ''
let #ptr = 1
let #end = 1
while #end > 0
let #end = instr($I_rec, $I_del, #ptr)
if #end > 0
let #len = #end - #ptr
if #len > 0
let PARmtx.PARfld (#idx) = substr($I_rec, #ptr, #len)
end-if
let #idx = #idx + 1
let #ptr = #end + 1
end-if
end-while
! Text Qualifiers Used (Each character must be tested)
else
let #len = length($I_rec)
let #txt = 0
let #idx = 0
let #pos = 1
let $data = ''
while #pos <= #len
let $char = substr($I_rec, #pos, 1)
if $char = $I_txt
let #txt = #txt + 1
else
if $char = $I_del
and mod(#txt, 2) = 0
let PARmtx.PARfld (#idx) = $data
let #idx = #idx + 1
let $data = ''
else
let $data = $data || $char
end-if
end-if
let #pos = #pos + 1
end-while
end-if
let #O_cols = #idx
end-procedure
!**********************************************************************
!* Parse Delimited Record (GET Field from Array) *
!**********************************************************************
!* *
!* INPUT: #I_idx - Index of Column to Retrieve *
!* OUTPUT: $O_data - Output Data String *
!* *
!* To de-activate this function: *
!* #define PARSE_Func_Remove *
!* *
!**********************************************************************
!* *
!* EXAMPLE: See PARSE-Array procedure for example. *
!* *
!**********************************************************************
begin-procedure PARSE-Func-Get(#I_idx, :$O_data)
let $O_data = PARmtx.PARfld (#I_idx)
end-procedure
#endif
!**********************************************************************
|

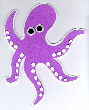 |
I would appreciate any feedback you may have on this site.
Send mail to tdelia@erols.com or click on the
Octopus. |

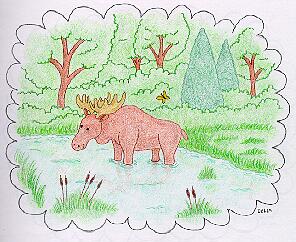 |
Please report any technical difficulties you may encounter to the address
above OR click on the Octopus. Thanks. |

NOTE - Use your Browsers BACK Button to return to prior page.

Tony DeLia - Updated October 5, 2000
|