| |
NOTE - Use your Browsers BACK Button to return to prior page or
CLICK here.
NXT-Func - Increment
Alpha-Numeric (Character) String |
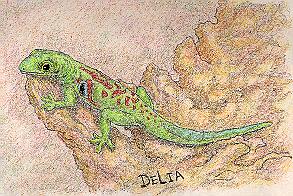 |
This function increments any character string... Consider the alphabet...
the letter 'A' + 1 equals 'B'... the letter 'Z' + 1 = 'BA'... 'BA' + 1 = 'BB'... Since the
alphabet is 26 characters it is actually a base26 calculation... the function detects the
base you're using and acts accordingly. I'll explain... for now check out my lizard...
click to enlarge. |

Let me preface this page by assuring everyone that I'm not insane... Also...
I spent more time formatting this page then I did creating the function/samples!
You can find the NXT-Func function in my custom SQR library TDFUNC.SQC

Let's look at the Binary Number System... there are 2 possible characters used to
represent any number... they are '0' and '1'... If we started out with '0' and added 1 we
would get '1'... add 1 again and we get '10'... then '11'... then '100'... we can
substitute any characters... say 'O' and 'X' where the 'O' represents ZERO and the'X'
represents ONE... still binary or base2... starting with 'O' the same results would be
displayed as... 'X', 'X0', 'XX' then 'XOO'... as you can see the characters have no
significance to the calculation... they have NO weighted value except the order of
precedence (we could have used 'X' to represent ZERO and 'O' for ONE)... |

NXT-Func - Input / Output
Parameters |
 | Input - Seed (Character Set in order - least to greatest) |
 | Input - Input String |
 | Output - Output String |
The seed is the character set for the particular number system:
Binary - '01', Octal - '01234567', Hexadecimal - '0123456789ABCDEF'
Alphabet - 'ABCDEFGHIJKLMNOPQRSTUVWXYZ', etc...
The number of characters in the seed determines the Base system used... in this case
the length of the seed variable... it's that simple!
|

EXAMPLE: let $I_seed = 'ABCD'
let $I_string = 'DAD'
do NXT-Func($I_seed, $I_string, $O_string)
show 'Next Value: ' $O_string
After the function executes $O_string equals 'DBA'... Why? Because this is a base4
operation... using the characters 'ABCD' as the seed... If we used '0123' instead the
example would use '303' as the input string and the incremented value would equal '310'...
internally the function tests for a character set overflow ('D' or '3' would bump past the
set) and adjusts by carrying one to the next digit position and 'zeroing' the current
position... Here's the function... |

!**********************************************************************
!* Next AlphaNumeric Increment - Array *
!**********************************************************************
!* *
!* INPUT: $I_seed - Character Set *
!* $I_string - Input String *
!* OUTPUT: $O_string - Output String (Next Increment) *
!* *
!* To de-activate this function: *
!* #define NXT_Func_Remove *
!* *
!**********************************************************************
!* *
!* EXAMPLE: do NXT-Func('01', '1111', $O_binary) *
!* do NXT-Func('0123456789ABCDEF', 'FF', $O_hex) *
!* do NXT-Func('ABCD', 'A', $O_alpha) *
!* *
!* RESULTS: $O_binary = '10000' *
!* $O_hex = '100' *
!* $O_alpha = 'B' *
!* *
!**********************************************************************
#ifndef NXT_Func_Remove
begin-procedure NXT-Array
create-array name=NXTmtx size=50 field=NXTval:number=1
let $NXTsw = 'Y'
end-procedure
!**********************************************************************
!* Next Alpha-Numeric String Increment *
!**********************************************************************
begin-procedure NXT-Func($I_seed, $I_string, :$O_string)
if $_NXTsw <> 'Y'
do NXT-Array
end-if
clear-array name=NXTmtx
let $O_string = ''
let #add = 1
let #top = length($I_seed)
let #len = length($I_string)
let #pos = #len
while #pos > 0
let #val = instr($I_seed, substr($I_string, #pos, 1), 1) + #add
if #val > #top
let #val = 1
else
let #add = 0
end-if
put #val into NXTmtx (#pos) NXTval
let #pos = #pos - 1
end-while
array-add #add to NXTmtx (0) NXTval
let #pos = 0
while #pos <= #len
let #val = NXTmtx.NXTval (#pos)
if #pos > 0
or #pos = 0
and #val > 1
let $O_string = $O_string || substr($I_seed, #val, 1)
end-if
let #pos = #pos + 1
end-while
end-procedure
#endif
|

Using the NXT-Func function in your program... |
Here's a simple demonstration... I've set a loop which executes 128 times... each pass
will increment several variables representing Binary, Octal, Hex and Alphabetic strings...
A multi-segment segment string is also included which is an invoice number in the format
NNNN-A... The invoice# is part numeric and part alpha... furthermore the alpha
portion is limited to A thru D... This means after '0001-D' comes '0002-A'... I'll get a
little tricky on this one... still it's fairly simple using the NXT-Func function... |
! FORWARDS
! Define Seeds (Character Sets)
let $I_dec = '0123456789'
let $I_bin = '01'
let $I_oct = '01234567'
let $I_hex = '0123456789ABCDEF'
let $I_alp = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'
let $I_inv1 = '0123456789'
let $I_inv2 = 'ABCD'
let $I_dec_val = '0'
let $I_bin_val = '0'
let $I_oct_val = '0'
let $I_hex_val = '0'
let $I_alp_val = 'A'
let $I_inv_val = '0000-D'
let #ctr = 1
while #ctr <= 128
! Decimal, Binary, Octal, Hexadecimal Increments
do NXT-Func($I_dec, $I_dec_val, $I_dec_val)
do NXT-Func($I_bin, $I_bin_val, $I_bin_val)
do NXT-Func($I_oct, $I_oct_val, $I_oct_val)
do NXT-Func($I_hex, $I_hex_val, $I_hex_val)
! Increment Multi-Segment Invoice No.
let $I1 = substr($I_inv_val,1,4)
let $I2 = substr($I_inv_val,6,1)
do NXT-Func($I_inv2, $I2, $I2)
if length($I2) > 1
let $I2 = substr($I2,2,1)
do NXT-Func($I_inv1, $I1, $I1)
end-if
let $I_inv_val = $I1 || '-' || $I2
do Print-Line
let #ctr = #ctr + 1
! Alphabetic Increment (A, B, C, D, etc... )
do NXT-Func($I_alp, $I_alp_val, $I_alp_val)
end-while
|

Sample Report Listing - Using the NXT-Func Function |
==================================================================================
Count Binary Octal Hex Decimal Alphabet Invoice
==================================================================================
1 1 1 1 1 A 0001-A
2 10 2 2 2 B 0001-B
3 11 3 3 3 C 0001-C
4 100 4 4 4 D 0001-D
5 101 5 5 5 E 0002-A
6 110 6 6 6 F 0002-B
7 111 7 7 7 G 0002-C
8 1000 10 8 8 H 0002-D
9 1001 11 9 9 I 0003-A
10 1010 12 A 10 J 0003-B
11 1011 13 B 11 K 0003-C
12 1100 14 C 12 L 0003-D
13 1101 15 D 13 M 0004-A
14 1110 16 E 14 N 0004-B
15 1111 17 F 15 O 0004-C
16 10000 20 10 16 P 0004-D
17 10001 21 11 17 Q 0005-A
18 10010 22 12 18 R 0005-B
19 10011 23 13 19 S 0005-C
20 10100 24 14 20 T 0005-D
21 10101 25 15 21 U 0006-A
22 10110 26 16 22 V 0006-B
23 10111 27 17 23 W 0006-C
24 11000 30 18 24 X 0006-D
25 11001 31 19 25 Y 0007-A
26 11010 32 1A 26 Z 0007-B
27 11011 33 1B 27 BA 0007-C
28 11100 34 1C 28 BB 0007-D
29 11101 35 1D 29 BC 0008-A
30 11110 36 1E 30 BD 0008-B
31 11111 37 1F 31 BE 0008-C
32 100000 40 20 32 BF 0008-D
33 100001 41 21 33 BG 0009-A
34 100010 42 22 34 BH 0009-B
35 100011 43 23 35 BI 0009-C
36 100100 44 24 36 BJ 0009-D
37 100101 45 25 37 BK 0010-A
38 100110 46 26 38 BL 0010-B
39 100111 47 27 39 BM 0010-C
40 101000 50 28 40 BN 0010-D
41 101001 51 29 41 BO 0011-A
42 101010 52 2A 42 BP 0011-B
43 101011 53 2B 43 BQ 0011-C
44 101100 54 2C 44 BR 0011-D
45 101101 55 2D 45 BS 0012-A
46 101110 56 2E 46 BT 0012-B
47 101111 57 2F 47 BU 0012-C
48 110000 60 30 48 BV 0012-D
49 110001 61 31 49 BW 0013-A
|

Decrementing a string... Simple... Reverse the seed!!! |
! BACKWARDS
! Reverse (Character Sets)
do REV-Func($I_dec, $I_dec)
do REV-Func($I_bin, $I_bin)
do REV-Func($I_oct, $I_oct)
do REV-Func($I_hex, $I_hex)
do REV-Func($I_alp, $I_alp)
do REV-Func($I_inv1, $I_inv1)
do REV-Func($I_inv2, $I_inv2)
do NXT-Func($I_alp, $I_alp_val, $I_alp_val) ! Alpha Offset
let #ctr = 128
while #ctr > 1
! Alphabetic Increment (Z, Y, X, W, etc... )
do NXT-Func($I_alp, $I_alp_val, $I_alp_val)
let #ctr = #ctr - 1
! Decimal, Binary, Octal, Hexadecimal Decrements
do NXT-Func($I_dec, $I_dec_val, $I_dec_val)
do NXT-Func($I_bin, $I_bin_val, $I_bin_val)
do NXT-Func($I_oct, $I_oct_val, $I_oct_val)
do NXT-Func($I_hex, $I_hex_val, $I_hex_val)
! Decrement Multi-Segment Invoice No.
let $I1 = substr($I_inv_val,1,4)
let $I2 = substr($I_inv_val,6,1)
do NXT-Func($I_inv2, $I2, $I2)
if length($I2) > 1
let $I2 = substr($I2,2,1)
do NXT-Func($I_inv1, $I1, $I1)
end-if
let $I_inv_val = $I1 || '-' || $I2
do Print-Line
end-while
|

Notice I threw in my REV-Func (also included in TDFUNC.SQC)... this takes an input
string and puts it in reverse order... Our seeds have been flipped... Binary is now '10',
Octal is '76543210', Hex is 'FEDCBA9876543210', etc. The NXT-Func will not perform any
differently... it still increments the string... but now the precedence of the characters
has changed causing a reverse effect! The sample report below picks up near the end of the
initial loop (incrementing)... now notice the effect after the count of 128 (separated by
a blank line)... All string values are decrementing properly... I love it when a plan
comes together... |

Sample Report Listing - Decrementing Portion |
==================================================================================
Count Binary Octal Hex Decimal Alphabet Invoice
==================================================================================
125 1111101 175 7D 125 EU 0032-A
126 1111110 176 7E 126 EV 0032-B
127 1111111 177 7F 127 EW 0032-C
128 10000000 200 80 128 EX 0032-D
127 1111111 177 7F 127 EW 0032-C
126 1111110 176 7E 126 EV 0032-B
125 1111101 175 7D 125 EU 0032-A
124 1111100 174 7C 124 ET 0031-D
123 1111011 173 7B 123 ES 0031-C
122 1111010 172 7A 122 ER 0031-B
121 1111001 171 79 121 EQ 0031-A
120 1111000 170 78 120 EP 0030-D
119 1110111 167 77 119 EO 0030-C
118 1110110 166 76 118 EN 0030-B
117 1110101 165 75 117 EM 0030-A
116 1110100 164 74 116 EL 0029-D
115 1110011 163 73 115 EK 0029-C
114 1110010 162 72 114 EJ 0029-B
113 1110001 161 71 113 EI 0029-A
112 1110000 160 70 112 EH 0028-D
111 1101111 157 6F 111 EG 0028-C
110 1101110 156 6E 110 EF 0028-B
|

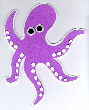 |
I would appreciate any feedback you may have on this site.
Send mail to tdelia@erols.com or click on the
Octopus. |

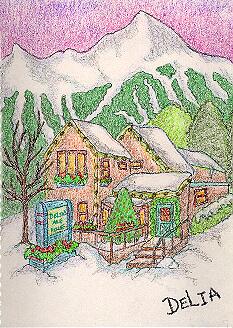 |
Please report any technical difficulties you may encounter to the address
above OR click on the Octopus. Thanks. |

NOTE - Use your Browsers BACK Button to return to prior page.

Tony DeLia - Updated March 03, 2000
|