SQR Techniques - File I/O
Validation Prompts |
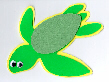 |
When developing new programs that require File I/O I gain alot of time
using this routine. Usage notes are included in the source code plus some notes on User
Prompts follow. |

TDFILIO.SQC - File I/O Validation Prompts |
!**********************************************************************
!* *
!* MODULE: TDFILIO.SQC *
!* AUTHOR: TONY DELIA. *
!* DATE: 05/20/97. *
!* SYSTEM: TD SQR UTILITY SERIES. *
!* DESC: FILE INPUT/OUTPUT VALIDATION PROMPTS. *
!* NOTE: THE FILES MUST BE OPENED IN THE SQR PROGRAM. *
!* VALIDATION ROUTINES OPEN THE FILE AND, IF VALID, *
!* ARE IMMEDIATELY CLOSED. IF INVALID, THE FILE MAY *
!* BE RE-ENTERED. THIS TYPE OF PROCESSING IS REQUIRED *
!* BECAUSE SQR DOES NOT ALLOW YOU TO PASS A LENGTH *
!* VARIABLE FOR THE OPEN STATEMENT (DEFAULT LEN = 1). *
!* #DEFINE STATEMENT COULD BE USED BUT THAT WOULD *
!* RESTRICT MULTIPLE INPUT/OUTPUT FILE PROCESSING *
!* (IF VARYING RECORD LENGTHS ARE REQUIRED). *
!* *
!**********************************************************************
!* *
!* REVISION: VARIABLE $td-x-msg ADDED TO OVERRIDE STANDARD *
!* INPUT PROMPTS. ROUTINE 'Alternate-TD-Prompt' MUST *
!* BE CODED IN YOUR SQR PROGRAM (MAY BE EMPTY). *
!* *
!**********************************************************************
!* *
!* USAGE: let #td-i-no = 1 *
!* let $td-x-msg = '' *
!* do Enter-TD-Input *
!* *
!* if #td-i-stat = 0 *
!* *
!* let #td-o-no = 2 *
!* let $td-o-warn = 'Y' *
!* let $td-x-msg = 'O1' ! Prompt Override *
!* do Enter-TD-Output *
!* *
!* if #td-o-stat = 0 *
!* . *
!* <Process Input/Output> *
!* . *
!* end-if *
!* end-if *
!* *
!* ! The code below overrides the standard prompts. *
!* *
!* begin-procedure Alternate-TD-Prompt *
!* *
!* evaluate $td-x-msg *
!* when = 'O1' *
!* input $td-x-file 'Enter Temporary File/Path' *
!* when-other *
!* input $td-x-file 'Enter File/Path' *
!* end-evaluate *
!* *
!* end-procedure *
!* *
!* #Include 'tdfilio.sqc' *
!* *
!**********************************************************************
!* *
!* PASS: #td-i-no => Input File Number *
!* #td-o-no => Output File Number *
!* $td-o-warn => Output File Overlay Warning *
!* $td-x-msg => Alternate Message Indicator *
!* *
!**********************************************************************
!* *
!* RETURNS: $td-i-file => Input File Name *
!* #td-i-stat => Input File Status *
!* $td-o-file => Output File Name *
!* #td-o-stat => Output File Status *
!* *
!**********************************************************************
!* *
!* LEGAL: CONFIDENTIALITY INFORMATION. *
!* *
!* This module is the original work of Tony DeLia. It *
!* can be considered ShareWare under the following *
!* conditions. *
!* *
!* A - The author's name (Tony DeLia) remains on any *
!* and all versions of this module. *
!* B - Any modifications must be clearly identified. *
!* C - A "vanilla" copy of this module must be kept *
!* alongside any revised versions. *
!* *
!**********************************************************************
!* *
!* WEBSITE: http://www.erols.com/tdelia/sqr.html *
!* *
!* Questions/Comments: tdelia@erols.com *
!* *
!**********************************************************************
!**********************************************************************
!* Enter TD Input *
!**********************************************************************
begin-procedure Enter-TD-Input
let $sw = 'Y'
while upper($sw) = 'Y'
let $sw = 'N'
if $td-x-msg = ''
input $td-i-file 'Enter INPUT path/filename' type=char
else
do Alternate-TD-Prompt
let $td-i-file = $td-x-file
end-if
open $td-i-file as #td-i-no
for-reading record=1 status=#td-i-stat
if #td-i-stat <> 0
input $sw maxlen=1 'OPEN Failed on INPUT. Retry? (Y/N)'
else
close #td-i-no
end-if
end-while
let $td-x-msg = ''
display ' '
display ' INPUT File: ' noline
display $td-i-file
display ' INPUT Status: ' noline
display #td-i-stat 99999
end-procedure
!**********************************************************************
!* Enter TD Output *
!**********************************************************************
begin-procedure Enter-TD-Output
let $sw = 'Y'
while upper($sw) = 'Y'
let $sw = 'N'
let $ov = 'Y'
if $td-x-msg = ''
input $td-o-file 'Enter OUTPUT path/filename' type=char
else
do Alternate-TD-Prompt
let $td-o-file = $td-x-file
end-if
if $td-o-warn = 'Y'
open $td-o-file as #td-o-no
for-reading record=1 status=#td-o-stat
if #td-o-stat = 0
close #td-o-no
input $ov maxlen=1 'Overlay Existing File? (Y/N)' type=char
uppercase $ov
end-if
end-if
if $ov = 'Y'
open $td-o-file as #td-o-no
for-writing record=1 status=#td-o-stat
if #td-o-stat <> 0
input $sw maxlen=1 'OPEN Failed on OUTPUT. Retry? (Y/N)'
else
close #td-o-no
end-if
else
let $sw = 'Y'
end-if
end-while
let $td-x-msg = ''
display ' '
display 'OUTPUT File: ' noline
display $td-o-file
display 'OUTPUT Status: ' noline
display #td-o-stat 99999
end-procedure
!**********************************************************************
|


INPUT:

 | Enter Input Path/Filename: |
Prompts for Input file name. The standard
prompt text may be overridden
using the $TD-X-MSG Alternate Message
Indicator.
 | OPEN failed on INPUT. Retry? (Y/N) |
If an invalid path/filename is entered this
error prompt appears. The user
has the option of re-entering the
path/filename or exiting.
The status indicator can be evaluated upon
returning to the
main 'calling' program. ( #TD-I-STAT = 0 if
successful ).

OUTPUT:

 | Enter Output Path/Filename: |
Prompts for Output file name. The standard
prompt text may be overridden
using the $TD-X-MSG Alternate Message
Indicator.
 | Overlay existing file? (Y/N): |
This prompt appears when the variable
$TD-O-WARN is set to 'Y'. This
warns the user that an output file already
exists. The user may decide to
use the file anyway or can enter a new
output filename.
 | OPEN failed on OUTPUT. Retry? (Y/N) |
If an invalid path/filename is entered this
error prompt appears. The user
has the option of re-entering the
path/filename or exiting.
The status indicator can be evaluated upon
returning to the
main 'calling' program. ( #TD-O-STAT = 0 if
successful ).

Once the Input or Output file has been entered and validated
it is the responsibility of the programmer to open the file(s) with the correct file
attributes (length/mode). This is mentioned in the usage notes in the source code. |

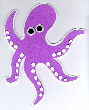 |
I would appreciate any feedback you may have on this site.
Send mail to tdelia@erols.com or click on the
Octopus. |
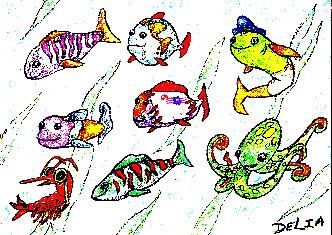 |
Please report any technical difficulties you may encounter to the address
above OR click on the Octopus. Thanks. |

Tony DeLia - Updated April 17, 1999
|